← View other
posts
Boss fight
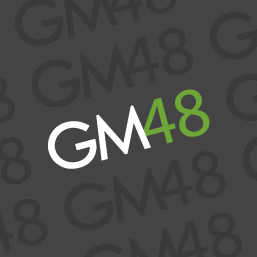
Lv. 1
class Shapoo: def init(self): self.name = "Shapoo" self.max_health = 1000 self.current_health = 1000 self.current_form = None self.sanity_level = 100 self.is_mimicking = False
def transform(self, player_character):
"""Shapeshifting ability to mimic player's abilities"""
self.current_form = player_character
self.is_mimicking = True
print(f"Shapoo transforms into a twisted version of {player_character.name}!")
def go_insane(self):
"""Increases unpredictability when sanity drops"""
self.sanity_level -= 25
if self.sanity_level <= 0:
self.spawn_explosive_clones()
def spawn_explosive_clones(self):
"""Creates clones that explode when health is low"""
clone_count = 3
print(f"Shapoo creates {clone_count} explosive clones!")
for _ in range(clone_count):
# Explosive clone logic
pass
def take_damage(self, damage, damage_type):
"""Custom damage handling"""
if damage_type == "gas":
# Vulnerable to gas attacks
damage *= 2
print("Gas attack is super effective against Shapoo!")
self.current_health -= damage
# Check for phase transition
if self.current_health <= 100: # 10% health
self.go_insane()
if self.current_health <= 0:
self.defeat()
def defeat(self):
"""Boss defeat logic"""
print("Shapoo lets out a final, distorted scream and collapses!")
return HeartTreasure()
class BossBattleArena: def init(self): self.environment = "Moving Train" self.current_carriage = 1 self.total_carriages = 5
def change_carriage(self):
"""Simulate train movement during battle"""
self.current_carriage += 1
print(f"Train shifts to carriage {self.current_carriage}")
# Add environmental challenges
if self.current_carriage % 2 == 0:
print("Carriage is unstable - increased dodge difficulty!")
class HeartTreasure: def init(self): self.name = "Cursed Heart" self.description = "A pulsing, dark red heart that seems to whisper ancient secrets"
def boss_battle(player): arena = BossBattleArena() shapoo = Shapoo()
print("BOSS ENCOUNTER: Shapoo on the Mystical Moving Train!")
while shapoo.current_health > 0:
# Battle loop with dynamic challenges
arena.change_carriage()
shapoo.transform(player)
# Player combat actions would go here
# For example:
# player_attack = get_player_attack()
# shapoo.take_damage(player_attack, attack_type)
treasure = shapoo.defeat()
return treasure